// main.cpp
int main (void)
{
//*******************************************************
// Initialization
//*******************************************************
IODIR0 |= RED_LED | GREEN_LED; //Set the Red and Green LED pins as outputs
IOSET0 = RED_LED | GREEN_LED;//Initially turn all of the LED's off
//*******************************************************
// Main Code
//*******************************************************
LCDInit();
LCDClear(BLACK);
LCDTestPixelSetOrder();
LCDPutStr("abcdefghijklm", 122, 122, RED, BLACK);
LCDPutStr("nopqrstuvwxyz", 122, 116, RED, BLACK);
LCDPutStr(" !&\"$%'()*+,-./", 122, 110, RED, BLACK);
LCDPutStr("0123456789:;?", 122, 104, RED, BLACK);
LCDPutStr("@[\\]^_`",122,98,RED,BLACK);
return 0;
}
// the following two functions exist in LCD_Driver.cpp, there also exists LCD_Driver.h
extern const unsigned char FONT5x5[91][5];
void LCDPutChar(char c, int x, int y, int fColor, int bColor)
{
int i,j;
unsigned int nCols;
unsigned int nRows;
unsigned int nBytes;
unsigned char PixelRow;
unsigned char Mask;
unsigned int Word0;
unsigned int Word1;
// get the nColumns, nRows and nBytes
// hard coded for now, not ideal
nCols = 6;
nRows = 5;
nBytes = 5;
// get pointer to the first byte of the desired character
PixelRow = FONT5x5[c-32][0];
// Row address set (command 0x2B)
LCDCommand(PASET);
LCDData(y);
LCDData(y + nRows - 1);
// Column address set (command 0x2A)
LCDCommand(CASET);
LCDData(x);
LCDData(x + nCols- 1);
// WRITE MEMORY
LCDCommand(RAMWR);
// loop on each row, working backwards from the bottom to the top
for (i = 0; i < nRows; i++)
{
// copy pixel row from font table and then decrement row
PixelRow = FONT5x5[c-32][i];
// loop on each pixel in the row (left to right)
// Note: we do two pixels each loop
Mask = 0x1;
//if (nCols%2 == 1)
//{
// nCols++;
//}
for (j = 0; j < nCols; j += 2) {
// if pixel bit set, use foreground color; else use the background color
// now get the pixel color for two successive pixels
if ((PixelRow & Mask) == 0)
Word0 = bColor;
else
Word0 = fColor;
Mask = Mask << 1;
if ((PixelRow & Mask) == 0)
Word1 = bColor;
else
Word1 = fColor;
Mask = Mask << 1;
// use this information to output three data bytes
LCDData((Word0 >> 4) & 0xFF);
LCDData(((Word0 & 0xF) << 4) | ((Word1 >> 8) & 0xF));
LCDData(Word1 & 0xFF);
}
}
// terminate the Write Memory command
LCDCommand(NOP);
}
void LCDPutStr(char *pString, int x, int y, int fColor, int bColor)
{
// loop until null-terminator is seen
while (*pString != 0x00)
{
// draw the character
LCDPutChar(*pString++, x, y, fColor, bColor);
// advance the x position
x = x - 6;
if (x > 131 || x < 0) break;
}
}
// this is in the file font.c (there exists a font.h too)
extern const unsigned char FONT5x5[91][5] =
{ 0x00, 0x00, 0x00, 0x00, 0x00, 0x10, 0x00, 0x10, 0x10, 0x10, // ' ' !
0x00, 0x00, 0x00, 0x0a, 0x0a, 0x0a, 0x1f, 0x0a, 0x1f, 0x0a, // " #
0x02, 0x07, 0x07, 0x07, 0x02, 0x10, 0x0a, 0x04, 0x0a, 0x01, // $ %
0x1e, 0x13, 0x1e, 0x12, 0x1e, 0x00, 0x00, 0x00, 0x08, 0x08, // & '
0x02, 0x04, 0x04, 0x04, 0x02, 0x08, 0x04, 0x04, 0x04, 0x08, // ( )
0x00, 0x0a, 0x04, 0x0e, 0x04, 0x00, 0x04, 0x0e, 0x04, 0x00, // * +
0x10, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x00, // , -
0x10, 0x00, 0x00, 0x00, 0x00, 0x10, 0x08, 0x04, 0x02, 0x01, // . /
0x1f, 0x11, 0x11, 0x11, 0x1f, 0x0e, 0x04, 0x04, 0x04, 0x0c, // 0 1
0x1f, 0x10, 0x1f, 0x01, 0x1e, 0x1f, 0x01, 0x0f, 0x01, 0x1f, // 2 3
0x02, 0x1f, 0x0a, 0x06, 0x02, 0x1e, 0x01, 0x1f, 0x10, 0x1f, // 4 5
0x1f, 0x11, 0x1f, 0x10, 0x0f, 0x08, 0x04, 0x02, 0x01, 0x1f, // 6 7
0x1f, 0x11, 0x1f, 0x11, 0x1f, 0x1f, 0x01, 0x1f, 0x11, 0x1f, // 8 9
0x00, 0x08, 0x00, 0x08, 0x00, 0x10, 0x08, 0x00, 0x08, 0x00, // : ;
0x00, 0x04, 0x08, 0x04, 0x00, 0x00, 0x0e, 0x00, 0x0e, 0x00, // < =
0x00, 0x04, 0x02, 0x04, 0x00, 0x04, 0x00, 0x06, 0x02, 0x0e, // > ?
0x1e, 0x1a, 0x1e, 0x1e, 0x00, 0x11, 0x11, 0x1f, 0x11, 0x1f, // @ A
0x1f, 0x11, 0x1e, 0x11, 0x1f, 0x1f, 0x11, 0x10, 0x11, 0x1f, // B C
0x1e, 0x11, 0x11, 0x11, 0x1e, 0x1f, 0x10, 0x1e, 0x10, 0x1f, // D E
0x10, 0x10, 0x1e, 0x10, 0x1f, 0x1f, 0x11, 0x13, 0x10, 0x1f, // F G
0x11, 0x11, 0x1f, 0x11, 0x11, 0x0e, 0x04, 0x04, 0x04, 0x0e, // H I
0x1f, 0x11, 0x01, 0x01, 0x01, 0x11, 0x12, 0x1c, 0x12, 0x11, // J K
0x1f, 0x10, 0x10, 0x10, 0x10, 0x15, 0x15, 0x15, 0x15, 0x1b, // L M
0x11, 0x13, 0x15, 0x19, 0x11, 0x1f, 0x11, 0x11, 0x11, 0x1f, // N O
0x10, 0x10, 0x1f, 0x11, 0x1f, 0x1f, 0x13, 0x11, 0x11, 0x1f, // P Q
0x11, 0x12, 0x1f, 0x11, 0x1f, 0x1f, 0x01, 0x1f, 0x10, 0x1f, // R S
0x04, 0x04, 0x04, 0x04, 0x1f, 0x1f, 0x11, 0x11, 0x11, 0x11, // T U
0x04, 0x0a, 0x11, 0x11, 0x11, 0x1b, 0x15, 0x15, 0x15, 0x15, // V W
0x11, 0x0a, 0x04, 0x0a, 0x11, 0x1f, 0x01, 0x1f, 0x11, 0x11, // X Y
0x1f, 0x08, 0x04, 0x02, 0x1f, 0x06, 0x04, 0x04, 0x04, 0x06, // Z [
0x01, 0x02, 0x04, 0x08, 0x10, 0x0c, 0x04, 0x04, 0x04, 0x0c, // \ ]
0x00, 0x00, 0x00, 0x0a, 0x04, 0x1f, 0x00, 0x00, 0x00, 0x00, // ^ _
0x00, 0x00, 0x00, 0x08, 0x10, 0x11, 0x11, 0x1f, 0x11, 0x1f, // ` A
0x1f, 0x11, 0x1e, 0x11, 0x1f, 0x1f, 0x11, 0x10, 0x11, 0x1f, // B C
0x1e, 0x11, 0x11, 0x11, 0x1e, 0x1f, 0x10, 0x1e, 0x10, 0x1f, // D E
0x10, 0x10, 0x1e, 0x10, 0x1f, 0x1f, 0x11, 0x13, 0x10, 0x1f, // F G
0x11, 0x11, 0x1f, 0x11, 0x11, 0x0e, 0x04, 0x04, 0x04, 0x0e, // H I
0x1f, 0x11, 0x01, 0x01, 0x01, 0x11, 0x12, 0x1c, 0x12, 0x11, // J K
0x1f, 0x10, 0x10, 0x10, 0x10, 0x15, 0x15, 0x15, 0x15, 0x1b, // L M
0x11, 0x13, 0x15, 0x19, 0x11, 0x1f, 0x11, 0x11, 0x11, 0x1f, // N O
0x10, 0x10, 0x1f, 0x11, 0x1f, 0x1f, 0x13, 0x11, 0x11, 0x1f, // P Q
0x11, 0x12, 0x1f, 0x11, 0x1f, 0x1f, 0x01, 0x1f, 0x10, 0x1f, // R S
0x04, 0x04, 0x04, 0x04, 0x1f, 0x1f, 0x11, 0x11, 0x11, 0x11, // T U
0x04, 0x0a, 0x11, 0x11, 0x11, 0x1b, 0x15, 0x15, 0x15, 0x15, // V W
0x11, 0x0a, 0x04, 0x0a, 0x11, 0x1f, 0x01, 0x1f, 0x11, 0x11, // X Y
0x1f, 0x08, 0x04, 0x02, 0x1f}; // Z
Below is the output to the driver additions outlined in the last few posts. Along with the font "bored" that I used as a guide.
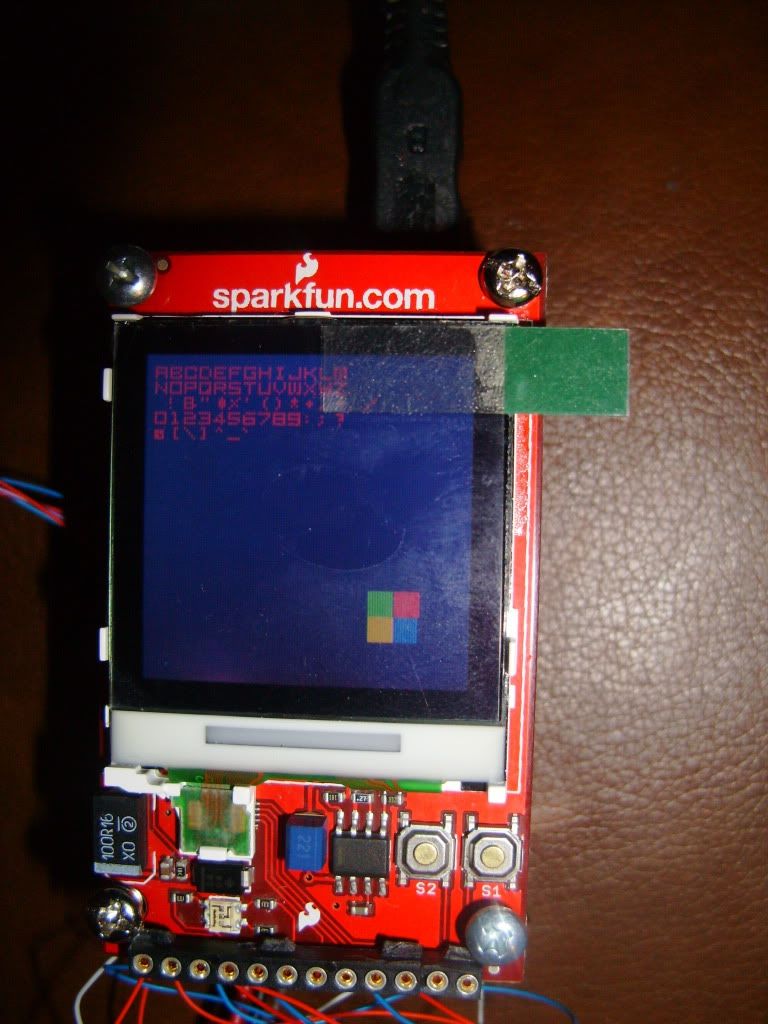
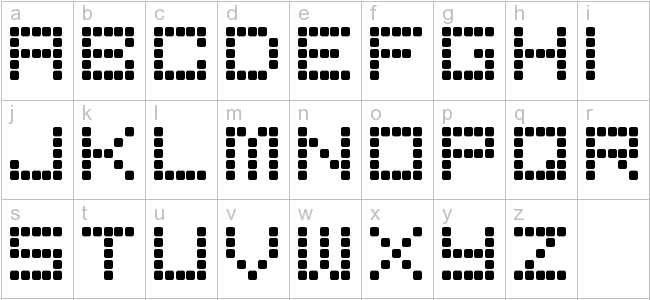
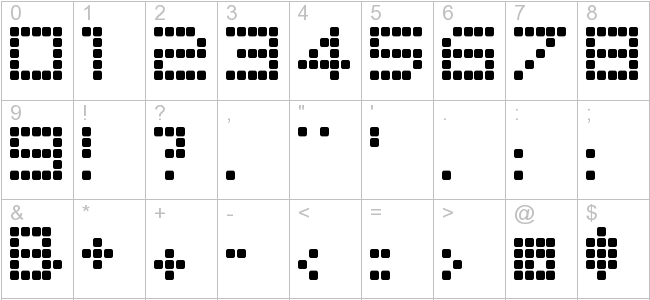
As can be seen the font is just enough to be functional, and that the colors at the bottom right seem to take different amounts of space. After testing a bit it can be shown that different colors seem to be aligned differently on the screen even thou they should occupy the same columns on the screen. Care will be needed to avoid graphics looking funny on the screen.
No comments:
Post a Comment